Argmax only supported for autoencoderkl
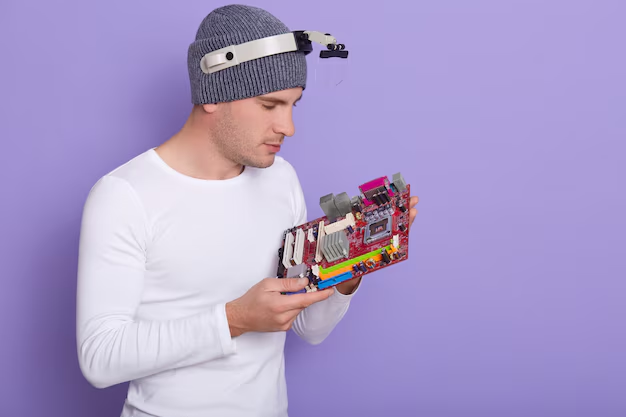
The error message “argmax only supported for autoencoderkl” typically arises in machine learning or deep learning tasks, particularly when working with specific models like autoencoders, especially AutoencoderKL (Kullback-Leibler). Here’s a breakdown of what it means and how you can address it.
Understanding the Error
AutoencoderKL is a type of autoencoder model that includes a variational aspect, using the Kullback-Leibler divergence to regularize its latent space. This ensures that the latent representations follow a distribution, often Gaussian, making them suitable for generative tasks.
The argmax
operation, which finds the index of the maximum value in a tensor or array, is usually used for discrete outputs (e.g., selecting the most probable class in classification tasks). However, in the context of AutoencoderKL, this operation might not be natively supported because the model deals with continuous latent spaces, where argmax is not meaningful.
Common Causes of the Error
- Inappropriate Operation: Applying
argmax
to the latent space or an intermediate layer that expects continuous values. - Model Mismatch: Using a decoder or a downstream process that assumes discrete outputs while the model provides continuous outputs.
- Incorrect Layer Usage: Misconfiguring the model to apply argmax where it isn’t supported.
How to Resolve the Error
1. Check Your Model’s Output
Ensure that the operation you’re applying aligns with the output type. For example:
- If the output is continuous, use operations like
softmax
orsigmoid
to process probabilities. - Use
argmax
only on discrete outputs, such as classification logits after a softmax activation.
2. Modify the Pipeline
If you’re working with an AutoencoderKL and need discrete outputs:
- Add a discrete sampling mechanism (e.g., Gumbel-Softmax) to approximate discrete distributions during training.
- Use post-processing to discretize outputs, if required, after generating the continuous data.
3. Debug the Code
Trace back to where argmax
is called. Ensure it’s applied only where supported, like categorical predictions. For latent spaces, consider using other operations suited for continuous data.
4. Update Dependencies
Sometimes, the error might occur due to mismatched library versions. Ensure you’re using the latest versions of libraries like PyTorch, TensorFlow, or the specific library that implements AutoencoderKL.
Example Fix
This ensures argmax
operates on probabilities rather than raw continuous latent space values.
Conclusion
The error “argmax only supported for autoencoderkl” emphasizes the importance of understanding your model’s outputs and applying appropriate operations. By aligning your pipeline with the model’s characteristics and ensuring the correct processing steps, you can avoid such errors and improve your implementation.